实验目标和实验内容:
1、掌握UI设计中的layout布局(约束布局)与基本控件(button、text、imageview等);
2、掌握复杂控件与adapter的使用
实验结果:(实验小结与结果截图)
3、功能说明与核心代码
这里主要是freagment+recycleradater的联合使用,基于之前做的实验
MainActivity布局文件
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<include layout="@layout/top" />
<FrameLayout
android:id="@+id/id_fragment"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:background="@drawable/singht">
</FrameLayout>
<include layout="@layout/buttom" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity:
这里主要进行fragment的加载
package com.example.mywechat;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.annotation.SuppressLint;
import android.app.Fragment;
import android.app.FragmentManager;
import android.app.FragmentTransaction;
import android.content.Intent;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.view.SurfaceControl;
import android.view.View;
import android.view.Window;
import android.os.Bundle;
import android.widget.Button;
import android.widget.ImageButton;
import android.widget.LinearLayout;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private Fragment fragment1= new weixinFragment();
private Fragment fragment2=new friendsFragment();
private Fragment fragment3= new linklistFragment();
private Fragment fragment4= new settingFragment();
private FragmentManager fm;
private LinearLayout mweixin;
private LinearLayout mfriends;
private LinearLayout mlinklist;
private LinearLayout msetting;
private ImageButton first1;
private ImageButton first2;
private ImageButton first3;
private ImageButton first4;
public RecyclerView recyclerView1;
public RecyclerView recyclerView2;
public RecyclerView recyclerView3;
public RecyclerView recyclerView4;
public void initView(){
mweixin=(LinearLayout) findViewById(R.id.id_weixin);
mfriends=(LinearLayout) findViewById(R.id.id_firends);
mlinklist=(LinearLayout) findViewById(R.id.id_link);
msetting=(LinearLayout) findViewById(R.id.id_set);
first1=(ImageButton)findViewById(R.id.img_weixin);
first2=(ImageButton)findViewById(R.id.img_friends);
first3=(ImageButton)findViewById(R.id.img_link);
first4=(ImageButton)findViewById(R.id.img_set);
recyclerView1=findViewById(R.id.newsrecyler);
recyclerView2=findViewById(R.id.friendsrecyler);
recyclerView3=findViewById(R.id.linkrecyclerview);
// recyclerView2=findViewById(R.id.settingrecycler);
}
public void initFragment(){
fm = getFragmentManager();
FragmentTransaction transaction=fm.beginTransaction();
transaction.add(R.id.id_fragment,fragment1);
transaction.add(R.id.id_fragment,fragment2);
transaction.add(R.id.id_fragment,fragment3);
transaction.add(R.id.id_fragment,fragment4);
transaction.commit();
}
public void hideFragment(FragmentTransaction transaction){
transaction.hide(fragment1);
transaction.hide(fragment2);
transaction.hide(fragment3);
transaction.hide(fragment4);
}
public void resetimg(){
first1.setImageResource(R.drawable.tab_weixin_normal);
first2.setImageResource(R.drawable.normal1);
first3.setImageResource(R.drawable.normal2);
first4.setImageResource(R.drawable.normal3);
}
public void selectFragment(int i){
FragmentTransaction transaction=fm.beginTransaction();
hideFragment(transaction);
resetimg();
switch (i){
case 0:
transaction.show(fragment1);
first1.setImageResource(R.drawable.tab_weixin_pressed);
break;
case 1:
transaction.show(fragment2);
first2.setImageResource(R.drawable.press1);
break;
case 2:
transaction.show(fragment3);
first3.setImageResource(R.drawable.press2);
break;
case 3:
transaction.show(fragment4);
first4.setImageResource(R.drawable.press3);
break;
default:
break;
}
transaction.commit();
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//requestWindowFeature(Window.FEATURE_NO_TITLE);
// initView();
//initEvent();
//initFragment();
//selectFragment(0);
setContentView(R.layout.activity_main);
initView();
initEvent();
initFragment();
selectFragment(0);
//initFragment();
}
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.img_weixin:
selectFragment(0);
break;
case R.id.img_friends:
selectFragment(1);
break;
case R.id.img_link:
selectFragment(2);
break;
case R.id.img_set:
selectFragment(3);
break;
}
}
public void initEvent(){
first1.setOnClickListener(this);
first2.setOnClickListener(this);
first3.setOnClickListener(this);
first4.setOnClickListener(this);
//msetting.setOnClickListener(this);
}
// @SuppressLint("ResourceType")
// @Override
// public boolean onCreateOptionsMenu(Menu menu) {
// MenuInflater menuInflater=getMenuInflater();
// menuInflater.inflate(R.menu.mymenu,menu);
// return true;
// //return super.onCreateOptionsMenu(menu);
// }
// @Override
// public boolean onOptionsItemSelected(@NonNull MenuItem item) {
// switch (item.getItemId()){
// case R.id.add:
// Toast.makeText(this,"添加了一个朋友",Toast.LENGTH_SHORT).show();
// break;
// case R.id.fabu:
// Toast.makeText(this,"发布了一个朋友圈动态",Toast.LENGTH_SHORT).show();
// break;
// case R.id.sao:
// Toast.makeText(this,"扫描了一个二维码",Toast.LENGTH_SHORT).show();
// break;
// }
// return super.onOptionsItemSelected(item);
// }
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
}
}
Fragment:
进行recycleradapter的相关布局编写
package com.example.mywechat;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.app.Fragment;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
/**
* A simple {@link Fragment} subclass.
* Use the {@link linklistFragment#newInstance} factory method to
* create an instance of this fragment.
*/
public class linklistFragment extends Fragment {
// TODO: Rename parameter arguments, choose names that match
// the fragment initialization parameters, e.g. ARG_ITEM_NUMBER
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
// TODO: Rename and change types of parameters
private String mParam1;
private String mParam2;
public RecyclerView recyclerView;
public View view;
public LinkAdapter linkAdapter;
public linklistFragment() {
// Required empty public constructor
}
/**
* Use this factory method to create a new instance of
* this fragment using the provided parameters.
*
* @param param1 Parameter 1.
* @param param2 Parameter 2.
* @return A new instance of fragment weixinFragment.
*/
// TODO: Rename and change types and number of parameters
public static linklistFragment newInstance(String param1, String param2) {
linklistFragment fragment = new linklistFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
// return inflater.inflate(R.layout.linklist, container, false);
view=inflater.inflate(R.layout.linklist,container,false);
recyclerView=view.findViewById(R.id.linkrecyclerview);
linkAdapter = new LinkAdapter(getActivity());//获取当前activity
recyclerView.setAdapter(linkAdapter);//设置适配器
recyclerView.setLayoutManager(new LinearLayoutManager(getActivity(),LinearLayoutManager.VERTICAL,false));//进行相应布局
return view;
}
}
Adapter:
进行recycler子类的编写并架子啊相应数据
package com.example.mywechat;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.recyclerview.widget.RecyclerView;
public class LinkAdapter extends RecyclerView.Adapter<LinkAdapter.ViewHolder>{
private LayoutInflater mInflater;
private String[] mTitles;
public LinkAdapter(Context context){
this.mInflater=LayoutInflater.from(context);//加载上下文
}
/**
* item显示类型
* @param parent
* @param viewType
* @return
*/
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view=mInflater.inflate(R.layout.linkitem,parent,false);//加载布局文件
//view.setBackgroundColor(Color.RED);
ViewHolder viewHolder=new ViewHolder(view);
return viewHolder;
}
/**
* 数据的绑定显示
* @param holder
* @param position
*/
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
//可进行相应数据的绑定
// holder.textView8.setText("张三");
// holder.textView9.setText("李四");
// holder.textView10.setText("王五");
// holder.textView11.setText("赵六");
// holder.textView12.setText("刘七");
}
@Override
public int getItemCount() {
return 9;//返回每个界面的数据条目
}
//自定义的ViewHolder,持有每个Item的的所有界面元素
public static class ViewHolder extends RecyclerView.ViewHolder {
//加载相应子类的控件
public TextView textView1;
public TextView textView2;
public TextView textView3;
public TextView textView4;
public TextView textView5;
public TextView textView6;
public TextView textView7;
public TextView textView8;
public TextView textView9;
public ImageView imageView1;
public ImageView imageView2;
public ImageView imageView3;
public ImageView imageView4;
public ImageView imageView5;
public ImageView imageView6;
public ImageView imageView7;
public ImageView imageView8;
public ImageView imageView9;
public ViewHolder(View view){
super(view);
textView1 = view.findViewById(R.id.linktext1);
textView2=view.findViewById(R.id.linktext2);
textView3=view.findViewById(R.id.linktext3);
textView4=view.findViewById(R.id.linktext4);
textView5=view.findViewById(R.id.linktext5);
textView6 = view.findViewById(R.id.linktext6);
textView7=view.findViewById(R.id.linktext7);
textView8=view.findViewById(R.id.linktext8);
textView9=view.findViewById(R.id.linktext9);
imageView1=view.findViewById(R.id.linkimage1);
imageView2=view.findViewById(R.id.linkimage2);
imageView3=view.findViewById(R.id.linkimage3);
imageView4=view.findViewById(R.id.linkimage4);
imageView5=view.findViewById(R.id.linkimage5);
imageView6=view.findViewById(R.id.linkimage6);
imageView7=view.findViewById(R.id.linkimage7);
imageView8=view.findViewById(R.id.linkimage8);
imageView9=view.findViewById(R.id.linkimage9);
}
}
}
4、结果截图
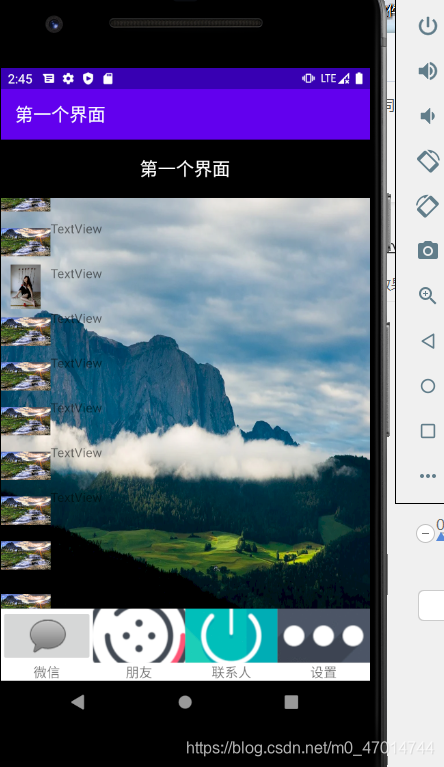
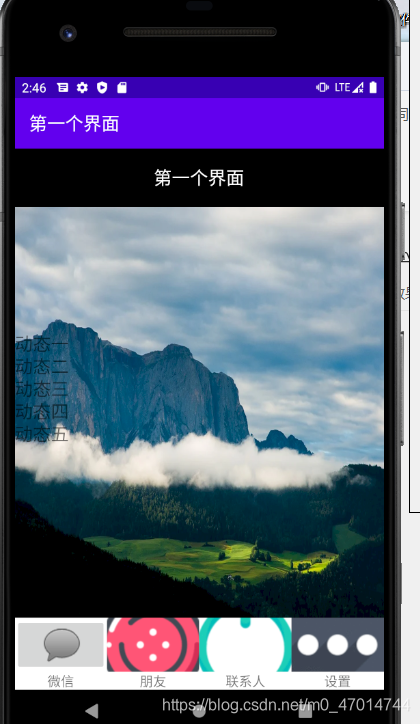